Changing Boot Screen¶
Boot Screen appears to WiPhone display during boot-up process. It gets stored in program memory, so it needs extra bit of work to change it compare to changing the WiPhone background image. Resoultion of Selected image should be kept 240x320 to correctly display on-screen boot image. user will need to convert desired image into array of data that will be placed in code’s header file. After making the required changes, modified firmware will be uploaded to WiPhone which will display the new boot time screen image.
How to Do this in Firmware¶
There is a SplashApp in GUI.cpp
which is used to draw the boot
screen of WiPhone. Code for this method is given as below:
void SplashApp::redrawScreen(bool redrawAll) {
// TODO: blink the LED from here
IconRle3 *icon;
uint8_t screen = screenNo % 4;
// Draw base icon
if (screen == 0) {
icon = new IconRle3(icon_splash_base, sizeof(icon_splash_base));
lcd.drawImage(*icon, 0, 0);
// Version
lcd.setTextColor(TFT_BLUE, TFT_WHITE);
lcd.setTextDatum(TL_DATUM);
lcd.setTextSize(1);
lcd.setTextFont(1);
lcd.drawString("Ver. " FIRMWARE_VERSION, 5, 310);
delete icon;
}
// Draw wifi icon
if (screen == 0) {
icon = new IconRle3(icon_splash_1, sizeof(icon_splash_1));
} else if (screen == 1) {
icon = new IconRle3(icon_splash_2, sizeof(icon_splash_2));
} else if (screen == 2) {
icon = new IconRle3(icon_splash_3, sizeof(icon_splash_3));
} else {
icon = new IconRle3(icon_splash_4, sizeof(icon_splash_4));
}
lcd.drawImage(*icon, (lcd.width()-icon->width())>>1, 98);
delete icon;
}
To use the custom boot screen, you need to do this:
- Select the image for Boot screen of size max 240x320. Here we select below image to be used as boot screen.
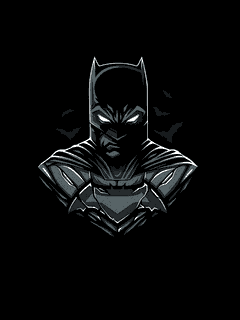
Run RLE3 script that will convert this image into 3-bit run-length encoded (RLE3) image format. Prerequisites for RLE3 scripts to run are:
- Python3
- OpenCV for Python3:
python3 -m pip install opencv-python
Input images supported by RLE3 script are:
- Indexed PNG images in RGB color mode (MUST not have more than 8 colors)
- Indexed PNG images in RGBA color mode (MUST not have more than 8 colors)
- Monochrome PNG images with transparency (will be quantized internally)
For more information related to RLE3 encoding script, see RLE3.py and README file at WiPhone\tools\RLE3\icons
You can use Photoshop or GIMP to convert your images into 8 colors only images.
In GIMP, you can do this from the image menubar through; Image → Mode → Indexed.
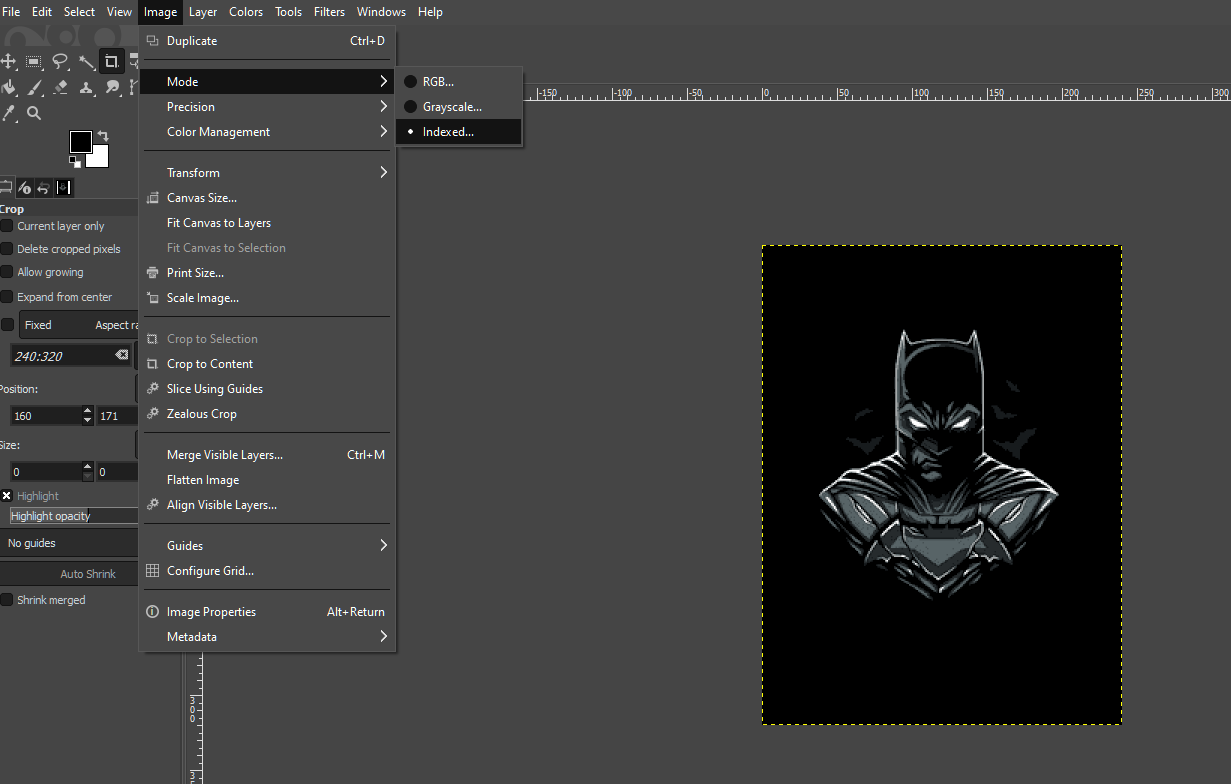
A dialog “Convert Image to Indexed Colors” opens up, there you can select maximum number of colors to 8.
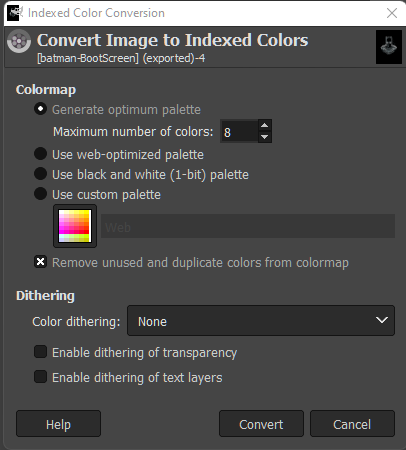
Place the desired image at \WiPhone\tools\RLE3\icons in WiPhone Firmware folder and run the command as below:
python3 RLE3.py BootScreen.png BootScreen.rle3
Now converts RLE3 image to constant C-arrays using to_c.py script.
python3 to_c.py BootScreen.rle3 BootScreen.h
You can place the resulting array data into header file icons.h located at WiPhone\src\assets
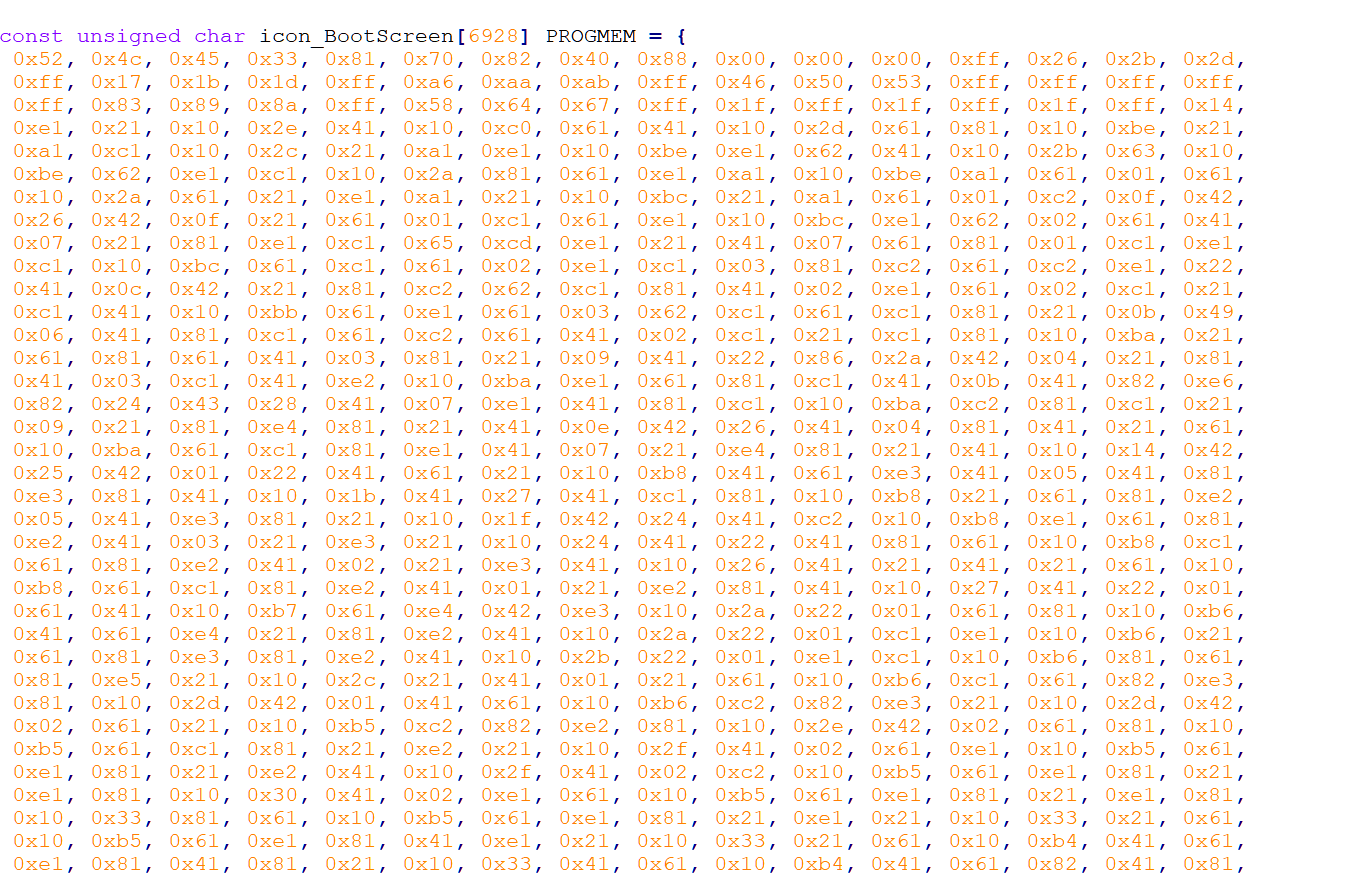
- Now modify the WiPhone Firmware to accommodate the changes.
In GUI.h SplashApp: Change the icon name for new boot screen as you saved in icons.h and upload the code to WiPhone.
Note: Comment out the WiFi Signal animation code in SplashApp for static boot screens and use the code as below :
void SplashApp::redrawScreen(bool redrawAll) {
// TODO: blink the LED from here
IconRle3 *icon;
uint8_t screen = screenNo % 4;
// Draw base icon
if (screen == 0) {
icon = new IconRle3(icon_BootScreen, sizeof(icon_BootScreen));
lcd.drawImage(*icon, 0, 0);
// Version
lcd.setTextColor(TFT_WHITE, TFT_BLACK);
lcd.setTextDatum(TL_DATUM);
lcd.setTextSize(1);
lcd.setTextFont(1);
lcd.drawString("Ver. " FIRMWARE_VERSION, 5, 310);
delete icon;
}
}
Compile and upload the firmware to WiPhone. On next Reboot, you will see the new bootup screen.
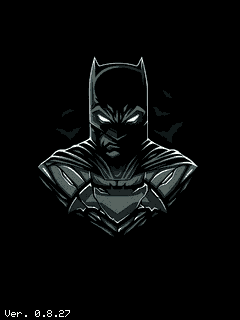
If you want to have a non-static bootup screen like we have wifi signal animation with default firmware of WiPhone at bootup. You can do this by displaying the series of images.
Convert any GIF to series of images and save as in a icons.h header files by same procedure as we have shown for static bootup screen.
Note: To process batch of all 8-color PNG pictures and produce their C array in single icons.h file, we have a script MAKE_ICONS.sh which can be used to process all images together
We used the GIF below and generated 20 static images to be used at bootup screen.
.. image:: media/Pv40RDc.gifModified the MAKE_ICONS.sh and processed all PNG images and generated their C array in icons.h. Then we can copy the data to icons.h located at WiPhone\src\assets
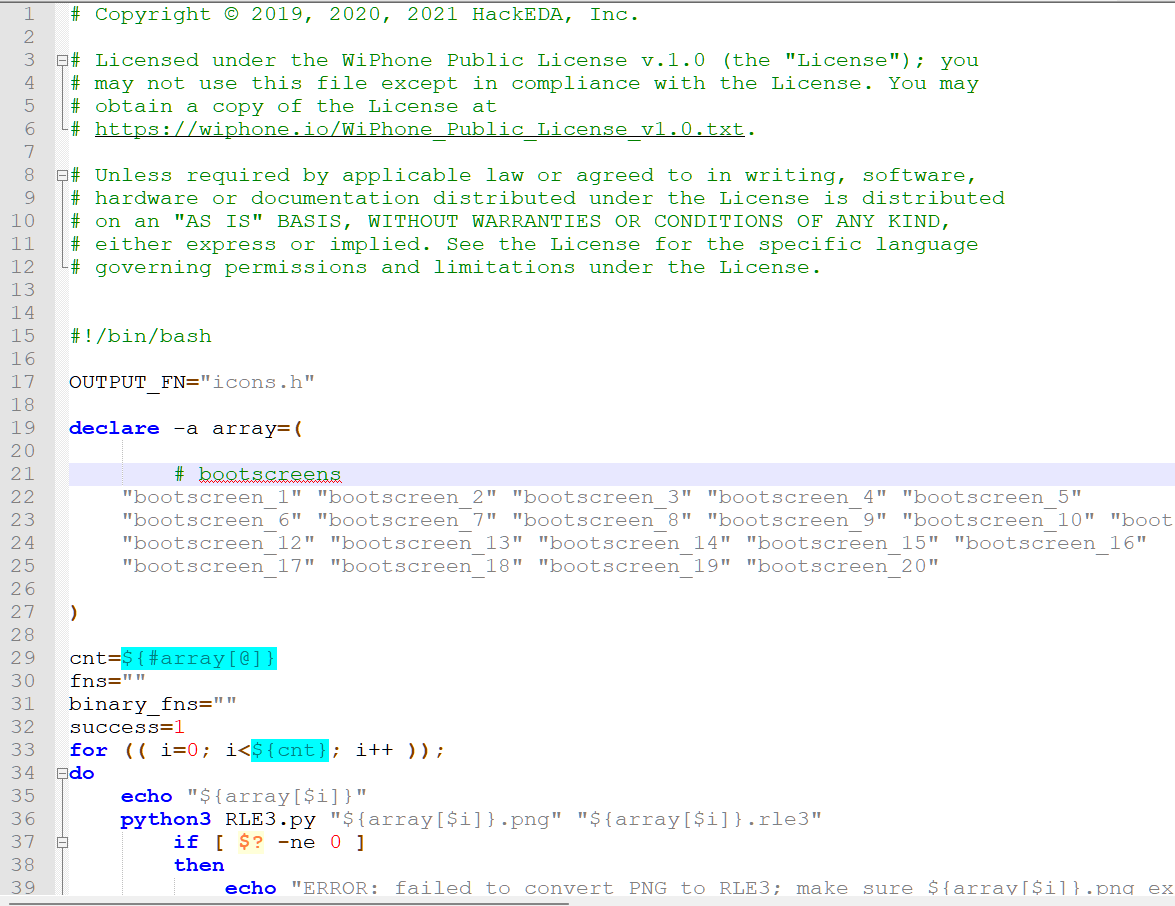
Modify the code to show the series of screens at bootup and upload to WiPhone.
SplashApp::~SplashApp() {}
appEventResult SplashApp::processEvent(EventType event) {
log_d("processEvent SplashApp");
if (LOGIC_BUTTON_BACK(event) || LOGIC_BUTTON_OK(event)) {
return EXIT_APP;
}
if (event==APP_TIMER_EVENT) {
return ++screenNo>=20 ? EXIT_APP : REDRAW_SCREEN;
}
return DO_NOTHING;
}
void SplashApp::redrawScreen(bool redrawAll) {
// TODO: blink the LED from here
IconRle3 *icon;
uint8_t screen = screenNo;
// Draw base icon
if (screen == 1) {
icon = new IconRle3(icon_bootscreen_1, sizeof(icon_bootscreen_1));
}
else if (screen == 2) {
icon = new IconRle3(icon_bootscreen_2, sizeof(icon_bootscreen_2));
} else if (screen == 3) {
icon = new IconRle3(icon_bootscreen_3, sizeof(icon_bootscreen_3));
} else if (screen == 4) {
icon = new IconRle3(icon_bootscreen_4, sizeof(icon_bootscreen_4));
} else if (screen == 5) {
icon = new IconRle3(icon_bootscreen_5, sizeof(icon_bootscreen_5));
} else if (screen == 6) {
icon = new IconRle3(icon_bootscreen_6, sizeof(icon_bootscreen_6));
} else if (screen == 7) {
icon = new IconRle3(icon_bootscreen_7, sizeof(icon_bootscreen_7));
} else if (screen == 8) {
icon = new IconRle3(icon_bootscreen_8, sizeof(icon_bootscreen_8));
} else if (screen == 9) {
icon = new IconRle3(icon_bootscreen_9, sizeof(icon_bootscreen_9));
} else if (screen == 10) {
icon = new IconRle3(icon_bootscreen_10, sizeof(icon_bootscreen_10));
} else if (screen == 11) {
icon = new IconRle3(icon_bootscreen_11, sizeof(icon_bootscreen_11));
} else if (screen == 12) {
icon = new IconRle3(icon_bootscreen_12, sizeof(icon_bootscreen_12));
} else if (screen == 13) {
icon = new IconRle3(icon_bootscreen_13, sizeof(icon_bootscreen_13));
} else if (screen == 14) {
icon = new IconRle3(icon_bootscreen_14, sizeof(icon_bootscreen_14));
} else if (screen == 15) {
icon = new IconRle3(icon_bootscreen_15, sizeof(icon_bootscreen_15));
} else if (screen == 16) {
icon = new IconRle3(icon_bootscreen_16, sizeof(icon_bootscreen_16));
} else if (screen == 17) {
icon = new IconRle3(icon_bootscreen_17, sizeof(icon_bootscreen_17));
} else if (screen == 18) {
icon = new IconRle3(icon_bootscreen_18, sizeof(icon_bootscreen_18));
} else if (screen == 19) {
icon = new IconRle3(icon_bootscreen_19, sizeof(icon_bootscreen_19));
} else {
icon = new IconRle3(icon_bootscreen_20, sizeof(icon_bootscreen_20));
}
lcd.drawImage(*icon, 0, 0);
delete icon;
}
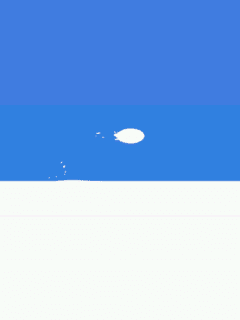