Battery Info App¶
The example app described here, shows how to extract the WiPhone's battery information and display it on-screen. The app also enables users to use this information in their other custom apps. Current Battery parameters such as; Charge percentage, voltages and charging status will be extracted and displayed on WiPhone's screen through firmware changes.
- After completing this Example, user will learn how to:
- Read the voltage, charge status (connected to USB or not) and SOC (state of charge) information from battery circuit
- Write battery status information on label widgets
How it works in WiPhone Interface¶
This example can be found within the WiPhone Menu Section; Tools -> Development -> Software Examples -> Battery Info. Once you click open that App, an activity screen titled "Battery Info App" will open, where you see current values against SOC, Battery voltage and charging status Placed in Center of display. Pressing "Back" button at bottom right corner exits the App. WiPhone's screenshot for this App activity is given below.
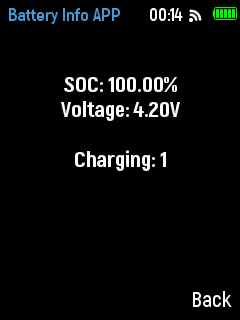
BatteryExample App
Implementation in Firmware¶
Identify Major Files & Functions¶
- GUI.h
- GUI.cpp
- processEvent (EventType event)
- redrawScreen (bool redrawAll)
- typedef enum ActionID : uint16_t {}
- GUIMenuItem menu[xx] PROGMEM = {}
- void GUI::enterApp (ActionID_t app)
- state.battsoc app event type returns current status of battery
- state.battVoltage shows battery voltages
- state.usbConnected determines whether usb charging is connected
Declaring a unique name for your app in GUI.h file¶
Search for "typedef enum ActionID" method in GUI.h and put your app name "GUI_APP_BatteryApp" as Action ID at designated place`.
Adding App Class into Header File¶
Insert following class code into GUI.h file.
GUI.h:
class BatteryApp : public WindowedApp {
public:
BatteryApp( LCD& disp, ControlState& state, HeaderWidget* header, FooterWidget* footer);
virtual ~BatteryApp();
ActionID_t getId() { return GUI_APP_BatteryApp; };
appEventResult processEvent(EventType event);
void redrawScreen(bool redrawAll = false);
void showInfo(String showString);
protected:
bool anyKeyPressed;
bool screenInited = false;
LabelWidget* label1;
LabelWidget* label2;
LabelWidget* label3;
};
Instantiating the App¶
Before defining the App classes, search for GUI::enterApp(ActionID_t app) in GUI.cpp file and append below line in switch statement to instantiate the App object.
case GUI_APP_BatteryApp:
// NOTE : THIS IS AN EXAMPLE BATTERY APP
runningApp = new BatteryApp(*screen, state, header, footer);
break;
The designated place to add above line within the switch statement in code can be found at enterApp Method in GUI.cpp.
Define App Constructor¶
A constructor method that we already instantiated in step above, needs to be defined first in GUI.CPP. After that, other related functions to follow in order to fully implement our example App. Let’s add constructor function for class BatteryApp.
GUI.cpp
BatteryApp::BatteryApp( LCD& lcd, ControlState& state, HeaderWidget* header, FooterWidget* footer)
: WindowedApp(lcd, state, header, footer) {
header->setTitle("Battery Info APP");
footer->setButtons(NULL, "Back");
String show_voltage = "V: "+String(gui.state.battVoltage); // Shows Voltage
String show_connection = "U: "+String(gui.state.usbConnected); // Shows USB connected or not
String show_battery = "C: "+String(gui.state.battSoc); // Shows Battery is being charged or not
label1 = new LabelWidget(0, (lcd.height()-fonts[AKROBAT_EXTRABOLD_22]->height())/2.5, lcd.width(), fonts[AKROBAT_EXTRABOLD_22]->height(),
show_battery.c_str(), WHITE, BLACK, fonts[AKROBAT_EXTRABOLD_22], LabelWidget::CENTER);
label2 = new LabelWidget(0, (lcd.height()-fonts[AKROBAT_EXTRABOLD_22]->height())/2, lcd.width(), fonts[AKROBAT_EXTRABOLD_22]->height(),
show_voltage.c_str(), WHITE, BLACK, fonts[AKROBAT_EXTRABOLD_22], LabelWidget::CENTER);
label3 = new LabelWidget(0, (lcd.height()-fonts[AKROBAT_EXTRABOLD_22]->height())/1.5, lcd.width(), fonts[AKROBAT_EXTRABOLD_22]->height(),
show_connection.c_str(), WHITE, BLACK, fonts[AKROBAT_EXTRABOLD_22], LabelWidget::CENTER);
}
Declare and Define other Methods¶
This segments will show us a way of adding source code of other functions/ methods used in Battery info Example. We declare and define these methods within the firmware code to fully implement our example App and subsequently put in place in GUI.cpp file. These functions are defined outside the BatteryApp class and thus presented here with scope resolution operator (::) as below:
- BatteryApp::~BatteryApp()
- BatteryApp::processEvent(EventType event);
- BatteryApp::redrawScreen(bool redrawAll);
BatteryApp::~BatteryApp(); This function defines BatteryApp's destructor to release memory after usage. All widgets must be registered and deleted by WiPhoneApp destructor.
BatteryApp::~BatteryApp() {
delete label1;
delete label2;
delete label3;
}
BatteryApp::processEvent(EventType event); This function defines the app events that fetch and display the required battery info on screen. To get the current status of the battery; use state.battsoc, to get battery voltages; use state.battVoltage, and to determine whether usb charging is connected or not; fetch state.usbConnected.
appEventResult BatteryApp::processEvent(EventType event) {
appEventResult res = DO_NOTHING;
if(label1 != NULL)
delete label1;
if(label2 != NULL)
delete label2;
if(label3 != NULL)
delete label3;
String show_voltage = "V: "+String(gui.state.battVoltage); // Shows Voltage
String show_connection = "U: "+String(gui.state.usbConnected); // Shows USB connected or not
String show_battery = "C: "+String(gui.state.battSoc); // Shows Battery is being charged or not
label1 = new LabelWidget(0, (lcd.height()-fonts[AKROBAT_EXTRABOLD_22]->height())/2.5, lcd.width(), fonts[AKROBAT_EXTRABOLD_22]->height(),
show_battery.c_str(), WHITE, BLACK, fonts[AKROBAT_EXTRABOLD_22], LabelWidget::CENTER);
label2 = new LabelWidget(0, (lcd.height()-fonts[AKROBAT_EXTRABOLD_22]->height())/2, lcd.width(), fonts[AKROBAT_EXTRABOLD_22]->height(),
show_voltage.c_str(), WHITE, BLACK, fonts[AKROBAT_EXTRABOLD_22], LabelWidget::CENTER);
label3 = new LabelWidget(0, (lcd.height()-fonts[AKROBAT_EXTRABOLD_22]->height())/1.5, lcd.width(), fonts[AKROBAT_EXTRABOLD_22]->height(),
show_connection.c_str(), WHITE, BLACK, fonts[AKROBAT_EXTRABOLD_22], LabelWidget::CENTER);
res |= REDRAW_SCREEN;
if (LOGIC_BUTTON_BACK(event) ) {
//ScreenShotTake();
return EXIT_APP;
}
return res;
}
BatteryApp::redrawScreen(bool redrawAll); This function is used when user wants to update any GUI for this app.
void BatteryApp::redrawScreen(bool redrawAll) {
if (!this->screenInited) {
redrawAll = true;
}
if (redrawAll) {
lcd.fillRect(0, header->height(), lcd.width(), lcd.height() - header->height() - footer->height(), TFT_BLACK);
}
((GUIWidget *) label1)->redraw(lcd);
((GUIWidget *) label2)->redraw(lcd);
((GUIWidget *) label3)->redraw(lcd);
screenInited = true;
}